It’s quite popular these days to interact with everything through mobile devices. Tapping, swiping, dragging things up and down, or even zooming in and out. All of these are possible with mobile devices.
Now, the problem arises, can all of these actions be done as well by desktop computers? Technically yes but most of the time no. Besides, it all depends on the capability of your display if it can do such actions like touch-screen.
But what if your desktop doesn’t have touch-screen features? Can you still drag or swipe? Maybe with the cursor instead?
100% yes! Thanks to Shopify Draggable, you can drag and drop web objects easily without too much programming.
So without further ado, let’s get started
What is Shopify Draggable
Shopify Draggable is a drag & drop library that allows developers to build interactive objects for users to drag from one place into another. For example, users can drag a product into the trash for deletion.
Shopify Draggable is also extensible which means if you have enough technical skills you can extend this library into something more useful. For instance, drag and popup or “drag and drop to see your prize!“.
Features
Unfortunately, Shopify Draggable is no longer being maintained by its original developers. However, this library could still give you the following features:
Installing Shopify Draggable
What we liked about Shopify draggable is that it could easily be installed in many ways. For example, if you are using Laravel for Shopify Development then you can just run the following code.
npm install @shopify/draggable --save
Code language: CSS (css)
If you’re using yarn then
yarn add @shopify/draggable
Code language: CSS (css)
On the other hand, if you want to use their CDN directly. You can use one of the following script tags
<!-- Entire bundle -->
<script src="https://cdn.jsdelivr.net/npm/@shopify/draggable@1.0.0-beta.8/lib/draggable.bundle.js"></script>
<!-- legacy bundle for older browsers (IE11) -->
<script src="https://cdn.jsdelivr.net/npm/@shopify/draggable@1.0.0-beta.8/lib/draggable.bundle.legacy.js"></script>
<!-- Draggable only -->
<script src="https://cdn.jsdelivr.net/npm/@shopify/draggable@1.0.0-beta.8/lib/draggable.js"></script>
<!-- Sortable only -->
<script src="https://cdn.jsdelivr.net/npm/@shopify/draggable@1.0.0-beta.8/lib/sortable.js"></script>
<!-- Droppable only -->
<script src="https://cdn.jsdelivr.net/npm/@shopify/draggable@1.0.0-beta.8/lib/droppable.js"></script>
<!-- Swappable only -->
<script src="https://cdn.jsdelivr.net/npm/@shopify/draggable@1.0.0-beta.8/lib/swappable.js"></script>
<!-- Plugins only -->
<script src="https://cdn.jsdelivr.net/npm/@shopify/draggable@1.0.0-beta.8/lib/plugins.js"></script>
Code language: HTML, XML (xml)
Browser Compatibility
If you are worried about this library not being compatible with all of the versions of the popular browsers then worry not! Because Shopify Draggable is compatible with:
Examples
Whenever you use Shopify Draggable, it is important to remember that draggable objects must be inside a container or element.
Also, we’re going to use this library without any frameworks which mean pure HTML is what we only needed. This way, it’ll be much easier for you to understand how this library works.
Don’t worry, we’ll be guiding you step by step on how to use Shopify Draggable.
First, let’s create an empty HTML file.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<style>
</style>
</head>
<body>
</body>
<script type="text/javascript">
</script>
</html>
Code language: HTML, XML (xml)
Next, let’s import the library using CDN.
Add the following line inside the head
tag.
<script src="https://cdn.jsdelivr.net/npm/@shopify/draggable@1.0.0-beta.2/lib/draggable.min.js"></script>
Code language: HTML, XML (xml)
Now that we have the library installed, let’s create the objects that we can drag.
Inside the body tag, copy the following code.
<div class="block">
<div class="droppable draggable-dropzone--occupied">
<div class="draggable"></div>
</div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
Code language: HTML, XML (xml)
In the code example above, we have declared three div classes and that is the block class, droppable class, and the draggable class.
Block Class
Take note that we called this “block” class because the above code, more specifically the div
tag has a class of block
. You can always change this class to whatever name you want as long as you specify that class in the javascript which we’ll do in a few moments.
Block class is where you can create all of the droppable objects. To simply explain this. All droppable elements must have their own parent block
class like what the example above has.
Droppable Class
This class is used for objects to be dropped into. So for example, you have one draggable object. You can drag this object into this section with droppable class.
Keep in mind that the size of draggable objects MUST be equal to the size of the droppable objects.
And again, you can rename this class to whatever you want as long as you specify this in your javascript.
Draggable Class
This class will make any elements draggable and can be dropped onto any element with the class of droppable
. Same with the rest, you can rename this class to whatever you want… and… of course specify it in the javascript. (We really love repeating ourselves, don’t we?)
Let’s continue
Inside the script tag, copy the following code.
const containers = document.querySelectorAll('.block')
const droppable = new Draggable.Droppable(containers, {
draggable: '.draggable',
droppable: '.droppable'
});
droppable.on('drag:start', () => console.log('drag:start'));
droppable.on('droppable:over', () => console.log('droppable:over'));
droppable.on('droppable:out', () => console.log('droppable:out'));
Code language: JavaScript (javascript)
The above code is written in Javascript ES6. So make sure you copy this code inside the script tags.
To explain the above code, we firstly declared a constant variable and we called it containers. Then we select all elements with the class of block
.
Currently, we have to two div elements with a class of block
so that’s what the variable will look for.
Next, we declare a new droppable object and target all the available blocks that can be used by draggable objects and then we assign which element is draggable and droppable.
After that, we initialize the events:
And then make a callback where we display in the console which event is currently happening.
Like we have mentioned before, it is important to give your objects their specified sizes to ensure that they will fit in their designated sections.
To do this, copy the following code inside the style tag.
.grid {
display: grid;
grid-template: 150px 150px 150px / 150px 150px 150px 150px;
grid-gap: 10px;
background-color: #ffffff;
padding: 10px;
}
.draggable {
background-color: #ff3946;
box-shadow: 10px 10px 10px #7777;
}
.draggable, .droppable.draggable-dropzone--occupied{
width: 100px;
height: 100px;
}
.droppable{
width: 100px;
height: 100px;
border: solid;
display: flex;
}
Code language: CSS (css)
The code above will only give our objects a little bit of style which doesn’t actually matter but since we need to give our objects a width then might as well give it a style.
If you have noticed, we have a new class at the top of this CSS which is the .grid
class.
This class does not matter as well, but this will organize our grid especially if we want to add more droppable sections on our page.
Let’s go back to our HTML and modify what’s inside the body tag:
<div class="grid">
<div class="block">
<div class="droppable draggable-dropzone--occupied">
<div class="draggable"></div>
</div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
</div>
Code language: HTML, XML (xml)
What we changed in our body tag is we simply added the grid class and then inserted our blocks inside of it.
We’re almost done!
Go save your file as index.html
and open that file in your browser.
You should see something like this:
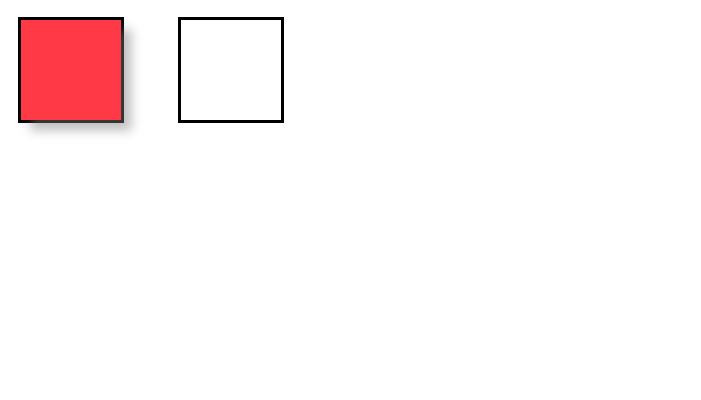
You may drag the red block into the empty block. You may also put it back into its place.
Now, what if you want to add more empty blocks for the draggable object?
The answer is very simple.
Just duplicate the block for the droppable object like below:
<div class="grid">
<div class="block">
<div class="droppable draggable-dropzone--occupied">
<div class="draggable"></div>
</div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
<div class="block">
<div class="droppable"></div>
</div>
</div>
Code language: HTML, XML (xml)
This will give you the following output:

Conclusion
There you go! You have finally developed a simple web page where an object can be dragged and dropped into different blocks.
What we really liked about this library is the fact that you don’t need to use jquery to make this library work. Not only that. It is ridiculously fast and lightweight.
I highly recommend you to experiment with this library. As we said before, this library is extensible meaning you can add more features to it.
If you want us to write more about Shopify Draggable library, let us know in the comments below and let us know as well your reactions by clicking one of the emojis below.
We love seeing your reactions 🙂
nice tutorial it’s very helpfull. please make video on shopify webhook event like order creation & cart page creation etc.
very nice tutorial but, can you make a little example using angular framework and draggable library because i have been found how to use it with angular and i didnt find anything