So you have finally set up your Shopify app. You have connected it to your web host. Now, you want to start working with Shopify API to display the products of the Shopify store.
In this tutorial, we’re going to take a look at how to display Shopify products using PHP.
If in case you ended up here and you don’t have any Shopify developer account, then we suggest reading our guide below to get started.
Video Tutorial
If you prefer watching tutorials over reading, you can watch the video version of this article below.
Getting Started
Create a new PHP file and name it products.php inside the file paste the following code:
<?php
require_once("inc/functions.php");
$requests = $_GET;
$hmac = $_GET['hmac'];
$serializeArray = serialize($requests);
$requests = array_diff_key($requests, array('hmac' => ''));
ksort($requests);
$token = PASTE YOUR GENERATED TOKEN HERE
$shop = PASTE YOUR SHOP URL HERE
Code language: PHP (php)
The code above will basically retrieve first the data of the Shopify store.
After getting all the data, assign the $_GET['hmac']
to a new variable $hmac
then serialize the $requests
variable. Then remove the key ‘hmac’ inside the array $requests.
Then we’ll sort the $requests
variable with ksort()
function.
Next, the following variables are important for making Shopify requests.
Remember that we generated a token when we installed the app in our store? Right now we’re going to use that, now paste the token in the value of $token
variable.
Note that we are not yet using the database and so it’s important to back up the access token in a file for reference.
Next, the $shop
variable. Paste the URL of the store that you are using for this app BUT without https and myshopify.com. Just the SUBDOMAIN.
Example:
https://this-is-the-subdomain.myshopify.com/
Using Collection API
$collectionList = shopify_call($token, $shop, "/admin/api/2020-07/custom_collections.json", array(), 'GET');
$collectionList = json_decode($collectionList['response'], JSON_PRETTY_PRINT);
$collection_id = $collectionList['custom_collections'][0]['id'];
$array = array("collection_id"=>$collection_id);
$collects = shopify_call($token, $shop, "/admin/api/2020-07/collects.json", $array, 'GET');
$collects = json_decode($collects['response'], JSON_PRETTY_PRINT);
Code language: PHP (php)
We’ll be using the function shopify_call()
to get the first collection ID that we needed for retrieving the products. You may skip this part if you want to display specific products from a collection ID.
To display specific products from a collection ID, you can use this code instead:
$collection_id = PASTE YOUR COLLECTION ID HERE
$array = array("collection_id"=>$collection_id);
$collects = shopify_call($token, $shop, "/admin/api/2020-07/collects.json", $array, 'GET');
$collects = json_decode($collects['response'], JSON_PRETTY_PRINT);
Code language: PHP (php)
Where can I get my collection id?

- Click Products
- Next, Collections
- Then click one of your desired collection.
- Check the URL
https://example.myshopify.com/admin/collections/1234567890
Code language: JavaScript (javascript)
The numerical value at the end of the URL above is the collection ID, copy that and paste it to your $collection_id
variable.
To explain, the code above will return an array that contains all the product IDs inside the collection ID that we used. Basically, it’s a JSON code but we decoded it and turn it into an array that’s why we use json_decode()
function.
Using Product API
foreach($collects as $collect){
foreach($collect as $key => $value){
$products = shopify_call($token, $shop, "/admin/api/2019-07/products/".$value['product_id'].".json", array(), 'GET');
$products = json_decode($products['response'], JSON_PRETTY_PRINT);
echo $products['product']['title'];
}
}
Code language: PHP (php)
The code above will display all the product’s name available inside the collection ID that we have used.
Output
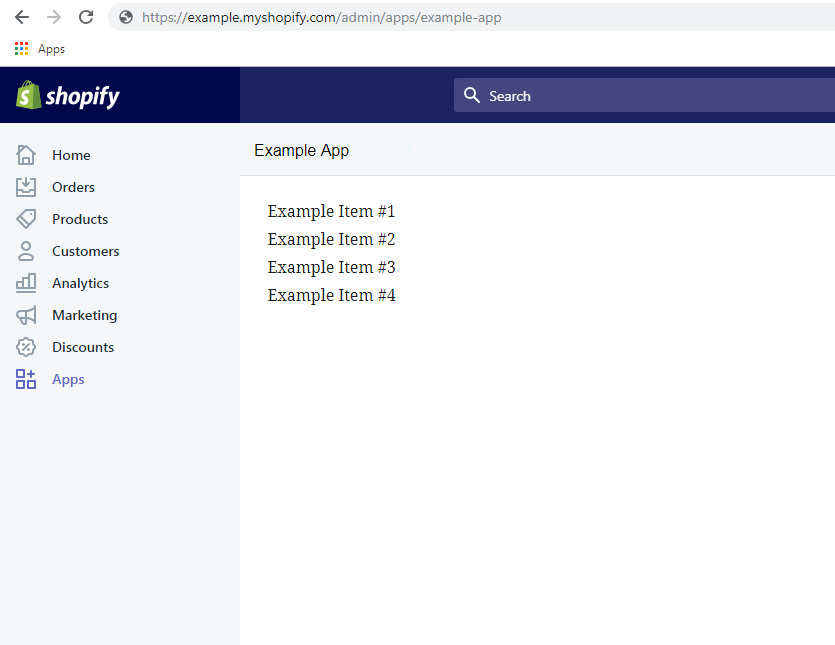
Congratulations! Now you have successfully displayed all the products that are available inside a certain collection. If you have concerns or questions, don’t hesitate to ask down in the comments.
How to run this products.php to show shopify products?
Hello Christian,
To run the PHP script to display the products from your shopify store, you have to make sure first that:
1.) You’re referencing ‘functions.php’
2.) You have replaced the values for the $token and $shop variables
3.) You are using the latest API versioning of Shopify which is 2019-04.
If you haven’t migrated your scripts to the latest version of API yet, I highly suggest you to read this article:
https://weeklyhow.com/building-shopify-apps-with-php-shopify-2019-04-update/
This will guide you to properly migrate your scripts from old API calls to latest API.
If you have more questions, please don’t hesitate to ask 🙂
Best Regards,
Bernard
Hello, I installed the app and all but when I try to run this products script I get “Warning: Invalid argument supplied for foreach() on line 24”. I did a print_r on the array and I got “(
[errors] => [API] Invalid API key or access token (unrecognized login or wrong password)
)” . What do you think is causing this issue?
Hello Aldin,
Have you checked if you’re using the right access token that you got from your install.php? Or is there no typo in your API key?
If the problem still persists, I suggest you to uninstall the app and re-install it again.
Thanks for reading!
Best Regards,
Bernard
Hello Bernard,
The same error is coming to my app. I tried storing access token in database but it is only storing blank in it.
I also tried uninstalling the app and re-installing it for 20 times but again and again the same error [errors] => [API] Invalid API key or access token (unrecognized login or wrong password) is coming up. Could you please help me with this.
Thanks
Anand
Hi Anand,
Thank you for reaching out.
What about the install uri?
Make sure it is formatted like this:
$install_url = "https://" . $shop . "/admin/oauth/authorize?client_id=" . $api_key . "&scope=" . $scopes . "&redirect_uri=" . urlencode($redirect_uri);
Best regards,
Bernard
Hi Bernard,
Thank You for your Response. However the problem could not be solved out even after trying what you said. I further tried different things and was able to find out that my generate_token. php file works well and even stores data in database, but the curl part is not working which is why it always leaves a blank in access_token in database. I have already enabled php curl in php extension.
Could you help me in this?
Thanks
Hi Anand,
Thank you for reaching out!
If the access_token is leaving empty value, that means there’s something wrong with your API key.
Check if there’s a typo, check your apps and your keys in your Developers account.
Check your store. Check your codes.
Also, you may try to reset the key access and secret key and try to replace it with a new one.
Don’t hesitate to tell me more details about the issue. We’d love to help.
Best regards,
Bernard
Hi Sir
i have same ERROR Array ( [errors] => [API] Invalid API key or access token (unrecognized login or wrong password) ) 1
ERROR URL — ” https://prnt.sc/zp8ups ”
i check my store. check my codes.
Could you help me in this?
After creating this product page and going back to the app in my shopify store I get an error saying “mysite” can’t be reached (the website used for the App URL).
I tried uninstalling and re-installing the app.
If I go directly to the product page created, I do see the products.
Any idea how to solve the connection issue in the app on shopify?
Hi Corey!
Thank you for reading!
Have you checked if you have an
index.php
file? that could be the problem.Best regards,
Bernard
Bernard,
Thank you for your response. I did not have an index.php file. However, I have now added that file and I am still getting the issue. I have uninstalled and reinstalled the app as well.
Any other areas I should look?
Thank you,
Corey
Hi Corey!
Have you tried renaming product.php to index.php?
That could do a trick. I believe
Also, there’s no need to uninstall and reinstall the app, as I believe this is the file issue.
Best regards,
Bernard
I have now tried renaming product.php to index.php. Unfortunately I get the same error. I inspected the element of the iframe. I got the redirect url and pasted that into my browser. I can confirm the redirect url works that the iframe is trying to load.
I am at a lost as to why the page won’t load in the app.
Hi Corey,
Thank you for reaching out about the issue.
If you want I could help you with the issue.
Send me an email at: bbpolidario@gmail.com
Thanks
I’m confused why you hard code the access token and site URL? Once in production we will not be able to hard code the user’s values. How do we go about storing/retrieving these values in production?
Thanks for your time.
Hi Anthony,
Thank you so much for taking the time to read our articles.
Yes, we have hardcoded the access token and the site URL in this particular tutorial for the reason that it’s a basic tutorial. We have written already how you can setup Shopify apps for database so merchants won’t have to deal with anything. Check out the article here: https://weeklyhow.com/how-to-save-shopify-access-token-in-database/
Cheers!
Thanks for the reply Bernard. I read that article, very helpful.
One question, how do you retrieve the token from the database? I assume we query where “shop url is equals to”, but how can i get the shop url dynamically to pass to the query?
Should we be using $_SERVER[‘HTTP_HOST’] or something? Or is there a shopify specific helper we can use to grab this current shop url?
Thanks again for your help.
Hi Anthony,
We’re really happy that we’re able to help you!
To answer your question, you can retrieve your access token, that’s right, through Shop URL in WHERE statement of MySQL query. You don’t have to use the
$_SERVER['HTTP_HOST']
, you can get the URL from$_GET
variable. You can useprint_r($_GET)
so you can see all the values you can get from$_GET
variable. For the Shop URL, it should be$_GET['shop']
.If you have more questions,
Let us know and we’d be really happy to help.
Cheers!
Bernard
Genius! Thank you Bernard, works perfect. On my way to building my first Shopify app thanks to your tutorials. Keep up the good work my friend!
Great article
I am concerned on the use of filezila.
Did you already make a connection to your domain?
Hello, I installed the app and all but when I try to run this products script I get “ a message “Could not resolve host: https”
How can i solved this ?